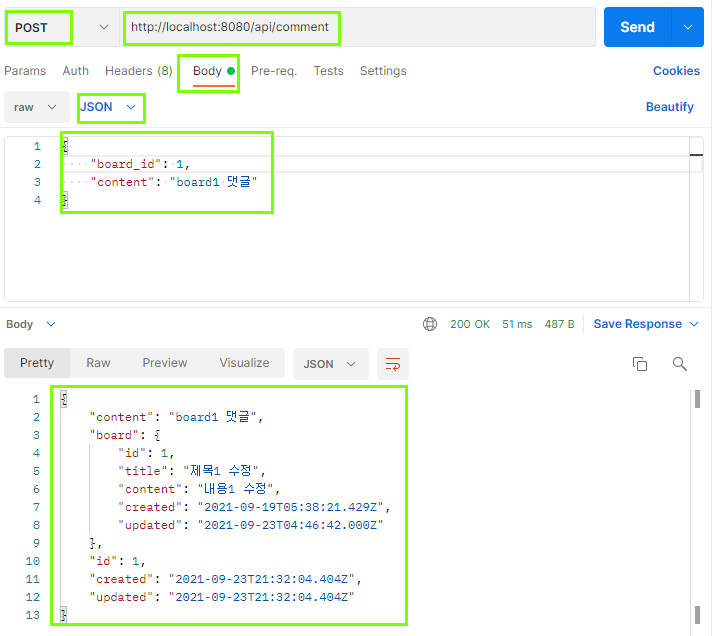
commet CRUD APIReact+REST API 게시판 구현/BE - TypeORM2021. 9. 24. 15:35
Table of Contents
What to do?
- 게시판 하단에 붙일 댓글 API를 만든다.
- comment 생성, 모두 보기, 하나보기(댓글 하나를 보는 화면은 없지만 댓글 생성후 생성된 댓글을 추가하기 위해서 필요), 수정, 삭제 이렇게 5개의 api를 만든다.
- https://duckgugong.tistory.com/226
API 설계
참고 https://eastflag.co.kr/fullstack/rest-with-nodejs/node-rest_board_post/ angular, react, vue 등의 최신프런트엔드로 풀스택 개발 백엔드는 spring boot, Node로 프런트엔드는 react, angular, vue 의 최..
duckgugong.tistory.com
- 우선 위 링크에 들어가서 기본적인 API 설계를 하자.
컨트롤 모듈화
- src 아래 controller 폴더 아래 CommentController.ts 파일을 생성한다.
comment 생성
- HTTP 메서드: POST
- url: /api/comment
- body에 json 형식으로 board_id와 content를 넣어서 POST 방식으로 전달해서 comment를 생성한다. board_id와 같은 id를 가진 board에 comment가 추가된다!
comment 모두 보기
- HTTP 메서드: GET
- url: /api/comment/list
- 쿼리 파라미터로 board_id를 넘겨서 해당 id를 가진 board의 모든 comment를 보여준다.
comment 하나 보기
- HTTP 메서드: GET
- url: /api/comment
- 쿼리 파라미터로 comment의 id를 넘겨서 해당 id의 comment 를 보여준다
comment 수정
- HTTP 메서드: PUT
- url: /api/comment
- body에 수정하고자 하는 commnet의 id와 content를 넘겨서 해당 id를 가진 comment의 content를 수정한다.
comment 삭제
- HTTP 메서드: DELETE
- url: /api/comment
- 쿼리 파라미터로 삭제하고자 하는 comment의 id를 넘겨서 해당 id를 가진 commemt를 삭제한다.
comment 생성시 주의사항
- comment 테이블의 foreign key인 board_id 는 one-to-many 관계에 의해서 자동생성된다.
- 엔티티에 board_id라는 컬럼이 존재하지 않으므로 이 컬럼을 사용할 수가 없고 board_id 대신에 연관된 board 정보를 넣어줘야 한다는것에 유념해야 한다.
comment 모두 보기
- join문으로 find 구문에서 relations 키를 이용하면 Board에 딸린 comment 를 모두 가져올 수 있다.
src/controller/CommentController.ts
import {Comment} from "../entity/Comment";
import {getConnection} from "typeorm";
import {Board} from "../entity/Board";
export class CommentController {
static addComment = async (req, res) => {
const {board_id, content} = req.body;
const board = await getConnection().getRepository(Board).findOne({id: board_id});
const comment = new Comment();
comment.content = content;
comment.board = board;
await getConnection().getRepository(Comment).save(comment);
res.send(comment);
}
static findAllComment = async (req, res) => {
const {board_id} = req.query;
// const boards = await getConnection().getRepository(Comment).find({ where: { board_id: board_id } });
const board = await getConnection().getRepository(Board)
.findOne({relations: ["comments"], where: {id: board_id}, order: {id: 'DESC'}});
res.send(board.comments);
}
static findOneComment = async (req, res) => {
const {id} = req.query;
const comment = await getConnection().getRepository(Comment).findOne({id});
console.log(comment);
res.send(comment);
}
static modifyComment = async (req, res) => {
const {id, content} = req.body;
const result = await getConnection().createQueryBuilder().update(Comment)
.set({content})
.where("id = :id", {id})
.execute();
res.send(result);
}
static removeComment = async (req, res) => {
const {id} = req.query;
const result = await getConnection()
.createQueryBuilder()
.delete()
.from(Comment)
.where("id = :id", {id})
.execute();
res.send(result);
}
}
라우팅 모듈화
- src 아래 router 폴더에 /api/comment 뒤의 서브라우팅을 처리해 줄 comment.ts 파일을 생성한 후, 각 API 기능별로 라우팅을 추가하자.
src/router/comments.ts
import {Router} from "express";
import {CommentController} from "../controller/CommentController";
const routes = Router();
routes.post('', CommentController.addComment);
routes.get('/list', CommentController.findAllComment);
routes.get('', CommentController.findOneComment);
routes.put('', CommentController.modifyComment);
routes.delete('', CommentController.removeComment);
export default routes;
- 그리고 /comment를 처리해 줄 부분을 src 아래 router 폴더에 있는 index.ts 파일에 추가하자.
import {Router} from "express";
...
// src/router/comment.ts 라우팅 모듈
import comment from "./comment";
...
const routes = Router();
...
routes.use('/comment', comment)
...
export default routes;
- id가 1인 board에 comment가 추가된 것을 확인할 수 있다!
- id가 1인 board인 comment들이 나타난다.
'React+REST API 게시판 구현 > BE - TypeORM' 카테고리의 다른 글
회원가입, 로그인 구현 (0) | 2021.09.24 |
---|---|
인증과 권한 설계 (0) | 2021.09.24 |
board에 image 추가하기 (0) | 2021.09.24 |
board 추가/보기(페이징)/수정/삭제 API (0) | 2021.09.20 |
컨트롤러, 라우팅 모듈화 (0) | 2021.09.19 |
@덕구공 :: Duck9s'
주니어 개발자에욤
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!