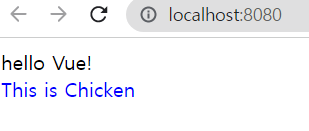
Vue의 component/data(State)/method/propsVue32022. 10. 13. 17:59
Table of Contents
Component 구성요소
- Vue의 컴포넌트는 .vue 라는 확장자를 가진 파일로 구성되어 있고 template, script, style 부분으로 나뉘어져 있다.
<template>
<div>hello Vue!</div>
</template>
<script>
export default {
name: "App",
};
</script>
<style>
</style>
- template 부분에는 실제 HTML을 작성하고 script 부분에는 컴포넌트 관련 로직들과 함수 및 state와 props 등에 관련한 내용을 작성하고 style 부분은 css를 작성한다.
- template 부분은 필수로 작성되어야 하며 script와 style 부분은 필요하지 않을 경우에는 삭제해도 된다.
- 그리고 template, script, style 부분은 아래처럼 외부 파일을 불러서 쓸 수도 있다.
<template src="a.html">
</template>
<script src="a.js">
</script>
<style src="a.css">
</style>
scoped style
- style 태그에 scoped라는 옵션을 추가하면 해당 컴포넌트에서만 적용되는 스타일을 의미한다.
- 아래처럼 style 태그에 scope 옵션을 추가하면 해당 컴포넌트의 chicken이라는 클래스에만 적용된다.
<style scoped>
.chicken {
color: blue;
}
</style>
자식 컴포넌트 불러오기
- 부모가 될 컴포넌트 부분의 script 태그 안에서 자식 컴포넌트를 import하고 export default의 components 프로퍼티에 불러온 컴포넌트를 적어주면 끝이다!
- 아래처럼 Chicken 컴포넌트를 만들고 App 컴포넌트에서 불러와보자!
- 단순히 import하고 export default의 component 옵션에 해당 컴포넌트의 이름만 넣어주면 된다!
Chicken.vue
<template>
<div class="chicken">This is Chicken</div>
</template>
<script>
</script>
export default {
data:{
}
}
<style scoped>
.chicken {
color: blue;
}
</style>
App.vue
<template>
<div>hello Vue!</div>
<Chicken />
</template>
<script>
import Chicken from "./components/Chicken.vue";
export default {
name: "App",
components: {
Chicken,
},
};
</script>
<style>
</style>
data(State)
- vue에서 state를 선언하는 방법은 매우 간단하다. export default문에 data 함수를 사용해서 원하는 값을 리턴해주기만 하면 된다!
- 이 data 속성 안에 있는 값들은 reactivity한 속성을 가진다
- Reactivity는 Vue.js의 특징 중 하나로 데이터의 변화를 라이브러리에서 감지하여 화면에 알아서 그려주는 것을 뜻한다.
- 아래처럼 state를 선언하면 name이라는 state에 "duck"이라는 값이 대입되고 height이라는 state에 180이라는 값이 대입된다!
export default {
name: "App",
data() {
return {
name: "duck",
height: 180,
};
},
};
- 또한, state 값을 View에 반영하기 위해 변수처럼 사용하려면 중괄호를 두번 중첩해서 감싸주면 해결된다!
- 그리고 JS 문법을 사용할 때도 중괄호를 두번 중첩해서 감싸서 사용하면 된다!
<template>
<div>{{ name }}</div>
<div>{{ height }}</div>
<div>{{ 1 + 1 }}</div>
<div>{{ true ? "hi" : "bye" }}</div>
</template>
- state를 선언하고 화면을 그려보자!
<template>
<div>{{ name }}</div>
<div>{{ height }}</div>
<div>{{ 1 + 1 }}</div>
<div>{{ true ? "hi" : "bye" }}</div>
</template>
<script>
export default {
name: "App",
data() {
return {
name: "duck",
height: 180,
};
},
};
</script>
<style>
</style>
++ 주의사항
- 만약 data 속성안에 아래처럼 return 문 안이 아닌 밖에서 this로 데이터를 할당하면 reactive 데이터가 아니게된다!
data(){
this.a = 1
return{
}
}
Method
- export default 구문 안에 methods라는 키워드로 컴포넌트에서 사용할 여러가지 method를 등록할 수 있다.
- 만약 method 안에서 state나 prop 값에 접근하고 싶다면 this 키워드를 사용해서 접근할 수 있다.
- 아래 예시를 통해 string, state, props를 각각 alert를 띄워보자!
부모 컴포넌트
- 부모 컴포넌트는 단지 name이라는 prop만 넘겨준다
<template>
<MethodTest name="duck" />
</template>
<script>
import MethodTest from "./components/MethodTest.vue";
export default {
components: { MethodTest },
name: "App",
};
</script>
PropsValidation
자식 컴포넌트
- 자식 컴포넌트에서 method 3개를 추가해서 각각 string, state, props 값의 alert를 띄운다!
<template>
<div>Method state&props test</div>
<div>
<button @click="alertA">A</button>
</div>
<div>
<button @click="alertState">alert state</button>
</div>
<div>
<button @click="alertProps">alert props</button>
</div>
</template>
<script>
export default {
data: function () {
return {
chicken: "Kyochon",
};
},
props: { name: String },
methods: {
alertA() {
alert("A");
},
alertState() {
alert(this.chicken);
},
alertProps() {
alert(this.name);
},
},
};
</script>
<style>
</style>
Props
- 자식 컴포넌트에게 props를 전달할 때 부모 컴포넌트에서 자식 컴포넌트를 불러오고 속성값을 추가하면 된다.
- 자식 컴포넌트에서는 부모 컴포넌트에서 받은 props를 사용하기 위해 export default 안의 props 속성에 사용할 props를 명시해주면 된다!
- 자식 컴포넌트에서 부모 컴포넌트에서 보내준 prop의 값은 변경이 불가능하다 read-only!!!
Static props
- 부모 컴포넌트에서 자식 컴포넌트로 string 값으로 props를 전달하는 방식이다.
부모 컴포넌트
<template>
<Chicken brand="BBQ" />
</template>
<script>
import Chicken from "./components/Chicken.vue";
export default {
name: "App",
components: {
Chicken,
},
};
</script>
<style>
</style>
자식 컴포넌트
- 아래처럼 props를 string의 array로 받아서 사용하거나
<template>
<div class="chicken">This is Chicken</div>
<div>brand is {{ brand }}</div>
</template>
<script>
export default {
props: ["brand"],
};
</script>
<style scoped>
.chicken {
color: blue;
}
</style>
- object 형식으로 props의 type과 함께 적어서 사용할 수 있다.
<template>
<div class="chicken">This is Chicken</div>
<div>brand is {{ brand }}</div>
</template>
<script>
export default {
props: {
brand: String,
},
};
</script>
<style scoped>
.chicken {
color: blue;
}
</style>
Dynamic props (v-bind)
- v-bind를 이용해 자바스크립트 표현식을 이용해서 string 값 이외에 다른 값(state, number, array, object 등등....)을 props로 전달할 수 있다.
- short-hand로 : 키워드를 붙여도 됨!
- 즉, 모든 값을 다 넘길 수 있다!
- v-bind를 사용하고 string 값을 사용할 땐 반드시 작은 따옴표(')로 감싸서 사용해야한다!!!!!
State
부모 컴포넌트
<template>
<Chicken :brand="brand" />
</template>
<script>
import Chicken from "./components/Chicken.vue";
export default {
data: function () {
return {
brand: "BBQ",
};
},
name: "App",
components: {
Chicken,
},
};
</script>
<style>
</style>
자식 컴포넌트
<template>
<div class="chicken">This is Chicken</div>
<div>brand is {{ brand }}</div>
</template>
<script>
export default {
props: { brand: String },
};
</script>
<style scoped>
.chicken {
color: blue;
}
</style>
String
- v-bind를 이용해 props를 건내줄 때 작은 따옴표(')로 감싸서 값을 전달할 수 있다.
부모 컴포넌트
<template>
<div>My name is {{ name }}</div>
<Chicken v-bind:brand="'BBQ'" />
</template>
<script>
import Chicken from "./components/Chicken.vue";
export default {
name: "App",
components: {
Chicken,
},
};
</script>
<style>
</style>
자식 컴포넌트
<template>
<div class="chicken">This is Chicken</div>
<div>brand is {{ brand }}</div>
</template>
<script>
export default {
props: { brand: String },
};
</script>
<style scoped>
.chicken {
color: blue;
}
</style>
Number
부모 컴포넌트
<template>
<Chicken v-bind:brand="4444" />
</template>
<script>
import Chicken from "./components/Chicken.vue";
export default {
name: "App",
components: {
Chicken,
},
};
</script>
<style>
</style>
자식 컴포넌트
<template>
<div class="chicken">This is Chicken</div>
<div>brand is {{ brand }}</div>
</template>
<script>
export default {
props: { brand: Number },
};
</script>
<style scoped>
.chicken {
color: blue;
}
</style>
Array
부모 컴포넌트
<template>
<!-- short hand -->
<Chicken :brand="['BBQ', 'Kyochon']" />
</template>
<script>
import Chicken from "./components/Chicken.vue";
export default {
name: "App",
components: {
Chicken,
},
};
</script>
<style>
</style>
자식 컴포넌트
<template>
<div class="chicken">This is Chicken</div>
<div>brand is {{ brand[0] }}</div>
<div>brand is {{ brand[1] }}</div>
</template>
<script>
export default {
props: { brand: Array },
};
</script>
<style scoped>
.chicken {
color: blue;
}
</style>
Object
- v-bind를 사용하고 string 값을 사용하려면 마찬가지로 작은 따옴표를 사용해야 한다!
부모 컴포넌트
<template>
<!-- short hand -->
<Chicken
:brand="{
name: 'BBQ',
taste: 'GOOD',
}"
/>
</template>
<script>
import Chicken from "./components/Chicken.vue";
export default {
name: "App",
components: {
Chicken,
},
};
</script>
<style>
</style>
자식 컴포넌트
<template>
<div class="chicken">This is Chicken</div>
<div>brand is {{ brand.name }}</div>
<div>it tastes {{ brand.taste }}</div>
</template>
<script>
export default {
props: { brand: Object },
};
</script>
<style scoped>
.chicken {
color: blue;
}
</style>
Props Validation
- Vue는 props에 대한 validation check를 할 수 있어서 개발자가 예기치못한 오류를 피할 수 있어서 이부분은 좋은 것 같다고 느낀다.
Type 체크
- 자식 컴포넌트에서 부모 컴포넌트에서 전달받을 props를 명시해서 type이 맞지 않다면 콘솔에서 warning이 나타난다.
부모 컴포넌트
<template>
<PropsValidation :a="1" />
</template>
<script>
import PropsValidation from "./components/PropsValidation.vue";
export default {
name: "App",
components: {
PropsValidation,
},
};
</script>
자식 컴포넌트
- 아래처럼 props를 객체의 key로 대입하고 바로 value로 타입을 지정해주거나
<template>
<div class="chicken">This is Chicken</div>
</template>
<script>
export default {
props: { brand: String },
};
</script>
<style scoped>
.chicken {
color: blue;
}
</style>
- 객체에 type이라는 키 값을 담아서 넣을 수 있다. 이런식으로 객체를 이용하면 type, value, default 등 다른 유효성을 같이 검사할 수 있다.
<template>
<div class="chicken">This is Chicken</div>
</template>
<script>
export default {
props: { brand: { type: String } },
};
</script>
<style scoped>
.chicken {
color: blue;
}
</style>
- 부모에서 Number로 넘겨 준 값에 대해 자식이 String이라고 받겠다 명시했기 때문에 개발자도구에서 warning을 띄워준다!
+++ 여러 type 허용
- array에 허용할 타입들을 넣어줘서 여러가지 type에 대해 허용을 해 줄 수 있다.
export default {
props: { brand: { type: [Number, String] } },
};
default value
- props에 default value를 지정해 줄 수 있다. 만약 부모 컴포넌트에서 값을 넘겨 주지 않았다면 이 값이 사용된다.
부모 컴포넌트
- 자식 컴포넌트를 불러오기만하고 props를 넘겨주지 않는 상태이다
<template>
<PropsValidation />
</template>
<script>
import PropsValidation from "./components/PropsValidation.vue";
export default {
name: "App",
components: {
PropsValidation,
},
};
</script>
자식 컴포넌트
- 부모 컴포넌트로부터 props를 넘겨받지 않았지만 default로 4444란 값을 가지고 있다.
<template>
<div>Props Validation</div>
<div>default value : {{ a }}</div>
</template>
<script>
export default {
props: {
a: { type: Number, default: 4444 },
},
};
</script>
- 부모에서 값을 넘겨주지 않았지만 default value인 4444가 화면에 출력된다!
+++ 주의사항!!!
- array나 object는 항상 default value가 함수로 return되어야 한다!_!
<script>
export default {
props: {
a: {
type: Object,
default: function () {
return { name: "duck" };
},
},
},
};
</script>
Custom Validation
- validator라는 키워드를 사용해서 직접 validation을 할 수 있다.
- 아래 예시는 person 객체의 name이라는 key 값이 duck이면 안되는 예시이다
부모 컴포넌트
<template>
<PropsValidation
:person="{
name: 'duck',
}"
/>
</template>
<script>
import PropsValidation from "./components/PropsValidation.vue";
export default {
name: "App",
components: {
PropsValidation,
},
};
</script>
PropsValidation
자식 컴포넌트
<template>
<div>Props Validation</div>
</template>
<script>
export default {
props: {
person: {
type: Object,
default: function () {
return { name: "duck" };
},
validator: function (initialValue) {
return initialValue.name !== "duck";
},
},
},
};
</script>
<style>
</style>
- name이 duck이기 때문에 validation warning이 발생했다.
'Vue3' 카테고리의 다른 글
Computed/Watch (0) | 2022.10.17 |
---|---|
Vue state 변화시키기 + (v-on/v-model/event) (0) | 2022.10.14 |
Vue 어플리케이션 구성요소 (0) | 2022.10.13 |
Vue-CLI → Vue 간단하게 실행하기 (0) | 2022.10.13 |
Vue란? (0) | 2022.10.13 |
@덕구공 :: Duck9s'
주니어 개발자에욤
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!